Passing arguments in functions means value given to function on which operation is to be performed.
There are three ways of passing arguments in functions.
- Call by value / Pass by Value
- Call by Reference / Pass by Reference
- Call by Address / Pass by Address
Call by value / Pass by value
In this type value is passed in function’s argument and operation is done on formal argument.
Any change made in formal argument does not effect the actual argument.
Q. Write a program to represent call by value for swapping of two variables.
#include<iostream.h>
#include<conio.h>
void swap(int,int);
void main()
{
clrscr();
int a,b;
a=10;
b=20;
cout<<”Before swapping a=”<<a<<”\tb=”<<b;
swap(a,b);
cout<<”\nAfter swapping in main a=”<<a<<”\tb=”<<b;
getch();
}
void swap(int x,int y)
{
int z;
z=x;
x=y;
y=z;
cout<<”\n After swapping in function a=”<<x<<”\tb=”<<y;
}
Output
Before swapping a=10 b=20
After swapping in function a= 20 b=10
After swapping in main a=10 b=20
OR (Run time)
#include<iostream.h>
#include<conio.h>
void swap(int,int);
void main()
{
clrscr();
int a,b;
cout<<”Enter value of a and b=”;
cin>>a>>b;
cout<<”Before swapping a=”<<a<<”\tb=”<<b;
swap(a,b);
cout<<”\nAfter swapping in main a=”<<a<<”\tb=”<<b;
getch();
}
void swap(int x,int y)
{
int z;
z=x;
x=y;
y=z;
cout<<”\n After swapping in function a=”<<x<<”\tb=”<<y;
}
Output
Enter value of a and b= 10
20
Before swapping a=10 b=20
After swapping in function a= 20 b=10
After swapping in main a=10 b=20
Call by Reference / Pass by Reference
In this type memory address is passed in function’s Argument Implicitly.
Any change made in formal argument is permanent.
Q. Write a program to represent call by Reference for swapping of two variables.
#include<iostream.h>
#include<conio.h>
void swap(int &,int &);
void main()
{
clrscr();
int a,b;
a=10;
b=20;
cout<<”Before swapping a=”<<a<<”\tb=”<<b;
swap(a,b);
cout<<”\nAfter swapping in main a=”<<a<<”\tb=”<<b;
getch();
}
void swap(int &x,int &y)
{
int z;
z=x;
x=y;
y=z;
cout<<”\n After swapping in function a=”<<x<<”\tb=”<<y;
}
Output
Before swapping a=10 b=20
After swapping in function a= 20 b=10
After swapping in main a=20 b=10
OR (Run time)
#include<iostream.h>
#include<conio.h>
void swap(int &,int &);
void main()
{
clrscr();
int a,b;
cout<<”Enter value of a and b=”;
cin>>a>>b;
cout<<”Before swapping a=”<<a<<”\tb=”<<b;
swap(a,b);
cout<<”\nAfter swapping in main a=”<<a<<”\tb=”<<b;
getch();
}
void swap(int &x,int &y)
{
int z;
z=x;
x=y;
y=z;
cout<<”\n After swapping in function a=”<<x<<”\tb=”<<y;
}
Output
Enter value of a and b= 10
20
Before swapping a=10 b=20
After swapping in function a= 20 b=10
After swapping in main a=20 b=10
Call by Address / Pass by Address
In this type memory address is passed in function’s Argument Explicitly.
Any change made in formal argument is permanent.
Q. Write a program to represent call by Address for swapping of two variables.
#include<iostream.h>
#include<conio.h>
void swap(int *, int *);
void main()
{
clrscr();
int a,b;
a=10;
b=20;
cout<<”Before swapping a=”<<a<<”\tb=”<<b;
swap(&a , &b);
cout<<”\nAfter swapping in main a=”<<a<<”\tb=”<<b;
getch();
}
void swap(int *x , int *y)
{
int *z;
*z=*x;
*x=*y;
*y=*z;
cout<<”\n After swapping in function a=”<<*x<<”\tb=”<<*y;
}
Output
Before swapping a=10 b=20
After swapping in function a= 20 b=10
After swapping in main a=20 b=10
OR (run time)
#include<iostream.h>
#include<conio.h>
void swap(int *, int *);
void main()
{
clrscr();
int a,b;
cout<<”Enter value of a and b=”;
cin>>a>>b;
cout<<”Before swapping a=”<<a<<”\tb=”<<b;
swap(&a , &b);
cout<<”\nAfter swapping in main a=”<<a<<”\tb=”<<b;
getch();
}
void swap(int *x , int *y)
{
int *z;
*z=*x;
*x=*y;
*y=*z;
cout<<”\n After swapping in function a=”<<*x<<”\tb=”<<*y;
}
Output
Enter value of a and b=10
20
Before swapping a=10 b=20
After swapping in function a= 20 b=10
After swapping in main a=20 b=10
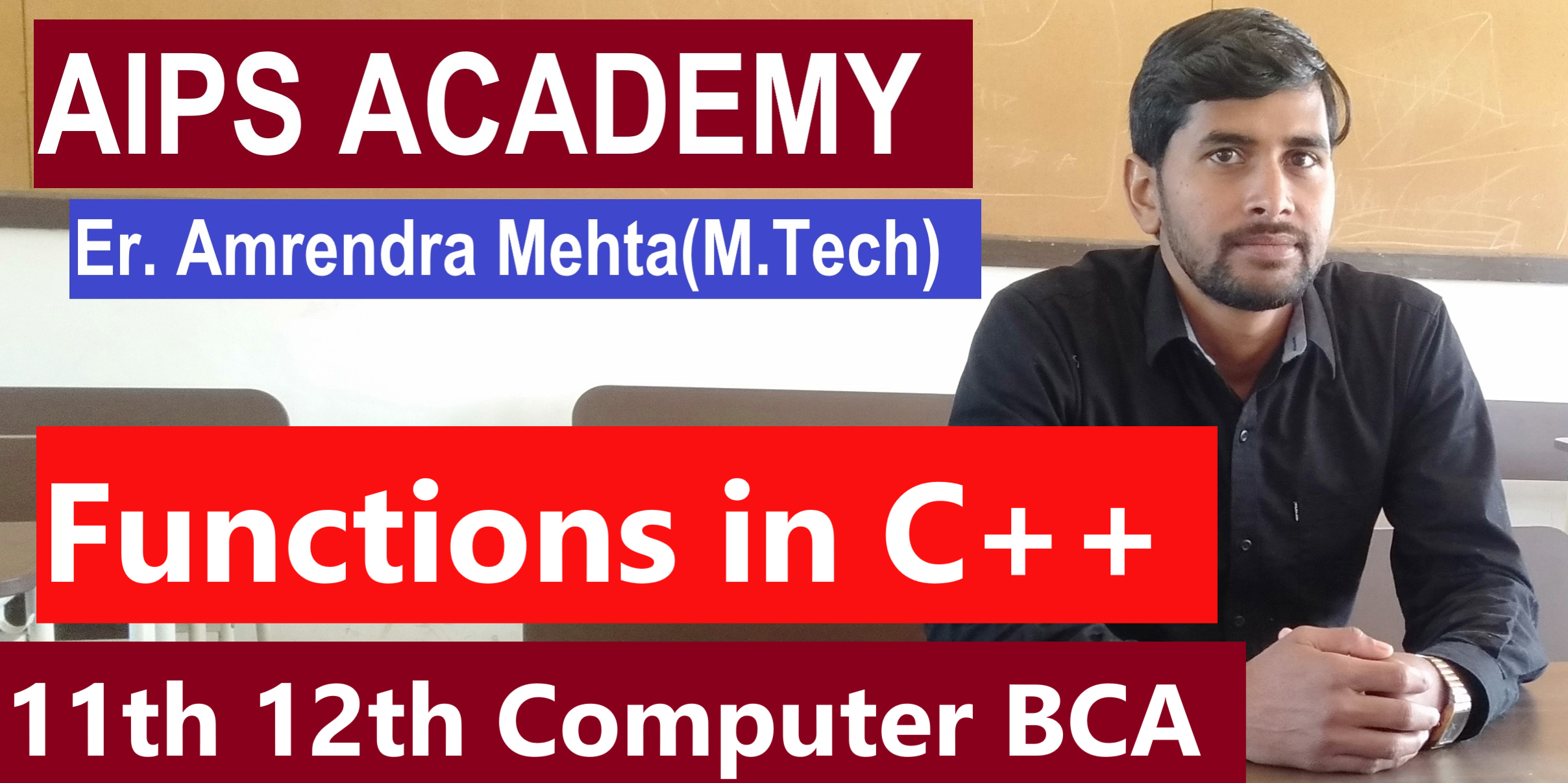